float sigmoid(float x) { //Logistic Function return 1.0 / (1 + exp(-x)); } float sigtime(int frameOffset, float persecond, boolean fast) { float t = (frameCount + frameOffset) / 60.0 * 2 * PI * persecond; float x = sin(t)*(fast ? 40 : 5); return sigmoid(x); } float triangularSaw(int offset, int framesA, int framesTrans) { //Returns 0, then increases linearly to 1, then stays at 1, then increases to 2 int totalCycle = (framesA + framesTrans) * 2; int currentFrame = (offset +frameCount) % totalCycle; if (currentFrame < framesA) return 0; else if (currentFrame < framesA + framesTrans) return map(currentFrame, framesA, framesA+framesTrans, 0, 1); else if (currentFrame < framesA + framesTrans + framesA) return 1; else return map(currentFrame, framesA + framesTrans + framesA, totalCycle, 1, 2); } void setup() { size(500, 500); smooth(); } void draw() { noStroke(); fill(255,50); rect(0,0,width,height); float dx = 80, dy = 80; for (int row = -5; row <= 5; row++) { for (int col = -5; col <= 5; col++) { int dtime = (int)map(dist(0, 0, col*dx, row*dy), 0, width, 0, -15); int br = (int)map(dist(0, 0, col*dx, row*dy), 0, width, 0, 255); float x = width / 2 + col * dx + (row % 2) * dx/2; float angle = triangularSaw(dtime+12/2*5, 54/2*5, 6/2*5)*PI; float y = height / 2 + row * dy + map(sigtime(dtime+0, .2, false), 0, 1, -100, 100); //println(y); textAlign(CENTER, CENTER); textSize(30); colorMode(HSB); fill(0, 0, br); pushMatrix(); translate(x, y); rotate(angle); translate(0,-5); //to get font rotation to better align around center text("dn", 0, 0); popMatrix(); } } }
Up and Dn
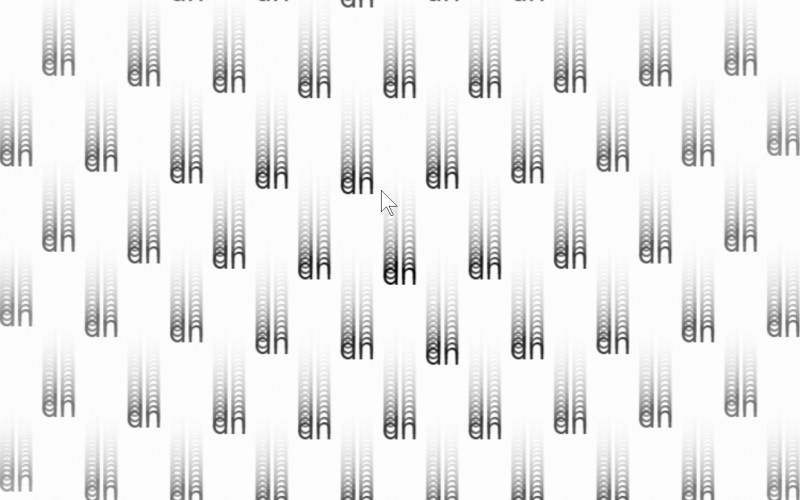
This animation was inspired by this reddit post.
Specifically, it makes use of the sigmoid (logistic) function, to simulate the up and down bouncing movement of the words. An additional “triangularSaw” function is used to time the rotations in sync with the movement. Finally, distance between the center word and other words is used to adjust brightness and add a slight offset to the timing of the movements.
[raw]
[/raw]
Code for the animation follows。 You can edit and run this code on the KTBYTE Coder