void setup() { size(800, 800); smooth(); } void draw() { background(255); float cx = width/2, cy=height/2, cw = width-20; pushMatrix(); translate(cx, cy); strokeWeight(cw/40); noFill(); stroke(100); ellipse(0, 0, cw, cw); if(!mousePressed) drawClockFaces(cw, 2); //Okay now let's draw the minute hand pushMatrix(); rotate(minute()/60.0*2*PI); strokeWeight(cw/70); stroke(100); line(0, 0, 0, -cw/2.0*2.8/4); popMatrix(); //Okay now let's draw the minute hand pushMatrix(); rotate((hour()/12.0+minute()/60.0/12.0)*2*PI); strokeWeight(cw/70); stroke(100); line(0, 0, 0, -cw/2.0*2.0/4); popMatrix(); rectMode(CENTER); rect(0, 0, cw/60, cw/60); textAlign(CENTER, TOP); fill(100); textSize(cw/15); text("TIME", 0, cw/20); popMatrix(); frameRate(5); //5 frames per second good enough after first frame } void drawClockFaces(float cw, int depth) { stroke(0); if (depth<0) return; for (int hour = 0; hour < 12; hour++) { float nextCx = (cw/2)/4.0*3.1; pushMatrix(); rotate(hour/12.0*2*PI-PI/2); translate(nextCx, 0); rotate(-hour/12.0*2*PI+PI/2); strokeWeight(1); float nextCw = cw/5; noFill(); textAlign(CENTER, TOP); fill(100); textSize(nextCw/15); text("TIME", 0, cw/200); strokeWeight(cw/100); line(0, 0, 0, -nextCw/2.0 * 6.5/10); pushMatrix(); rotate(2*PI*hour/12); line(0, 0, 0, -nextCw/2.0 * 4/10); popMatrix(); rectMode(CENTER); rect(0, 0, nextCw/60, nextCw/60); drawClockFaces(nextCw, depth-1); popMatrix(); } }
Clock, illustrating the idea of recursion
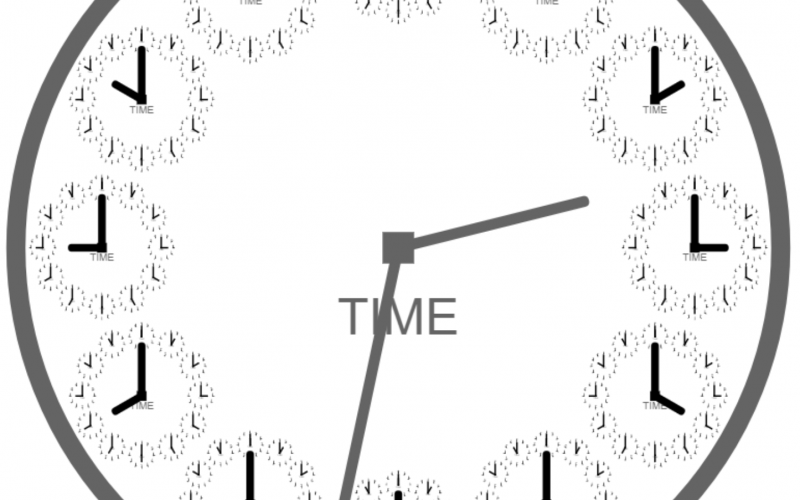
There is rarely a topic as confusing to students as recursion. Did you know that if you google “recursion”, google will suggest that you click on a link for recursion, and you can click forever and still end up in the same place? Recursion is the art of solving a problem by breaking the problem into smaller problems, which are then broken down into even smaller problems in the same way,.. etc. Of course, at some point, the programmer needs to define the solution to a ‘tiny’ problem without breaking it down any further, and we call these solutions the ‘base case’.
In the below sketch, instead of marking the hours with numbers, we’ve marked them with little clocks that represent the time, and each of those have little clocks that represent the time on them! Click on the sketch to disable the recursive function and only run the draw() function:
[raw]
[/raw]
Code for the recursive clock follows。 You can edit and run this code on the KTBYTE Coder
Your web site has exceptional material. I bookmarked the site