The Canadian Computing Competition (CCC) is a national-tier programming contest based on the same format as the International Olympiad in Informatics (IOI). This style is also shared by the Australian and US contests (AIO and USACO), making it part of a family of competitions where middle-to-high-school students can show their computer science skills and gain recognition with complex problem solving tasks. Out of those, the CCC is currently the most approachable in difficulty to start with. We recommend all students at least try out its practice problems to get started in this rewarding area of computer science!
The CCC is a great chance to demonstrate high-level achievement fairly quickly, and there is no prerequisite to try it. The next “main event” for the competition will be held on February 15, 2023, and we encourage all students near the KTBYTE offices or another participating school to give it a shot! If you’re only just getting started in your programming education and prefer not to try the main event just yet, that’s no problem – there are plenty of practice problems you can try out at your own pace.
Below, we’ll go over the details for how the CCC works, with a focus on how to try the practice problems available throughout the year. The main event coming up in February will work very similarly, but we’ll also remind participants of the specifics for participation as the date draws closer.
Problems, Divisions, and Awards
Each year the CCC releases a set of problems divided into two divisions of difficulty, Junior and Senior. Junior division problems are designed to be accessible for “any student with beginner programming skills”, while Senior division is for intermediate to advanced students. Within each division, the problems are given in increasing order of difficulty. Each division gets 5 problems to solve each year, although some problems overlap between divisions – for example, in 2022 the 4th Junior problem (J4) was also the 2nd Senior problem (S2). You can choose which division to participate in each year.
The progression of difficulties within any series of 5 Junior division problems is designed to make it easy to get started, but hard to get a top score.
- The first problem requires only basic programming and debugging ability, and learning the small “problem framework” for the program to operate correctly within the competition format – see below! The logical thinking requirement is equivalent to solving word problems in a math class, with only simple arithmetic or algebra needed for the program to get a correct answer. So this level is accessible for anyone who’s taken a typed Java class at KTBYTE! Even if you don’t try more difficult problems, you should try to solve one of these to get used to the competition format, using the website, and the overall feeling of this kind of contest.
- Middle problems aren’t too much harder, requiring work with slightly more programming concepts like loops and arrays, but very much within reach of [CORE 4] or [CORE 5] (formerly CS00 or CS01) KTBYTE students.
- Only the last problem or two in Junior division typically requires development of a more complex algorithm (problem-solving strategy), similar to a USACO Bronze level problem. And even the final problem provides the chance to get partial credit if your algorithm isn’t 100% perfect!
Each problem is worth 15 points, for a total of 75. The top 25% of students in each division receive a Certificate of Distinction, and high-scoring students are also listed on the Honor Roll for the year. The cutoff score that qualifies for recognition is lower in Senior division, but of course, the problems are much more challenging.
We recommend you start with Junior division for your first time participating. If you’ve done some competition training, you may find the first few problems very easy, but the last couple problems will still provide a challenge, and it’s a good way to ensure high chances of receiving immediate recognition for your skills. If you’ve done extensive training for this style of contest, such as for USACO Silver or Gold division, you could consider Senior division. However, most competitors can plan to start with Junior, and work on Senior division in a later year.
Practicing on the Website
Both practice problems and the actual competition can be accessed through http://cccgrader.com. You’ll need to register an account using a school code. If you’ll be taking the contest with us or just want to practice without doing the official event, please ask us our code, by emailing or calling the contact information at the bottom of our front page, http://www.ktbyte.com! Registering doesn’t mean you’re committed to doing the main February event, it just gives you access to the competition practice site, and the possibility of competing whenever you’re ready.
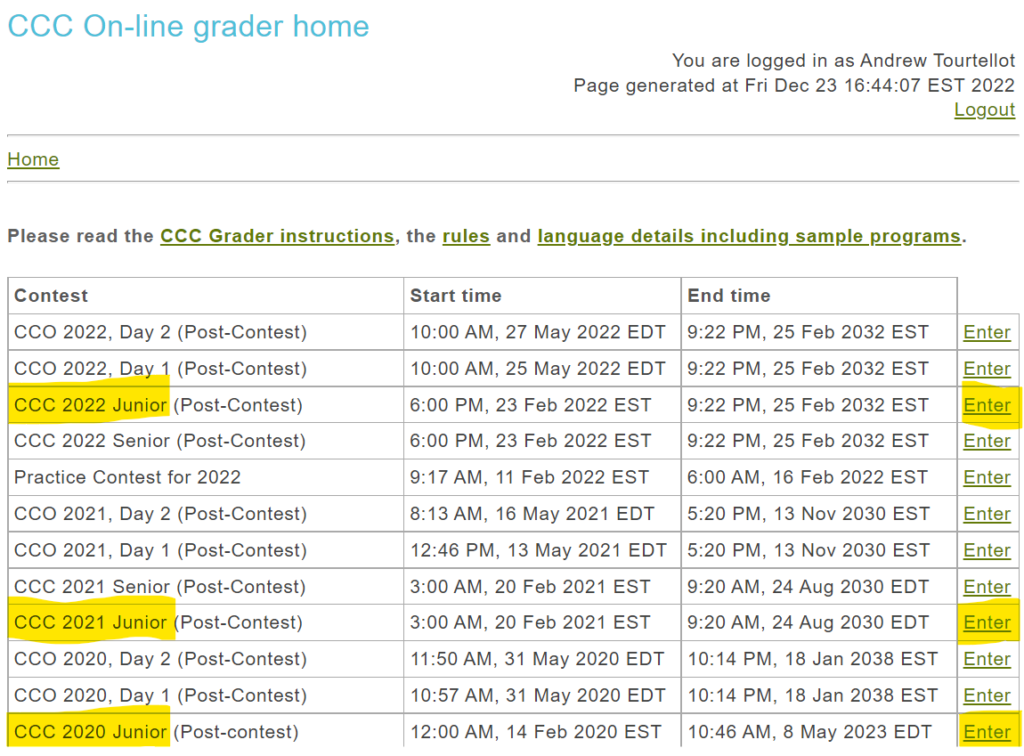
Once you’re logged in, you’ll see a long list of past contest rounds. You’ll want to focus on the CCC Junior division contests, and probably start with the most recent year or two. (The CCO is the invitational contest for the top 20 competitors in the country – no need to worry about that when you’re just starting!) Once you’ve picked a round, click Enter to access the problems.
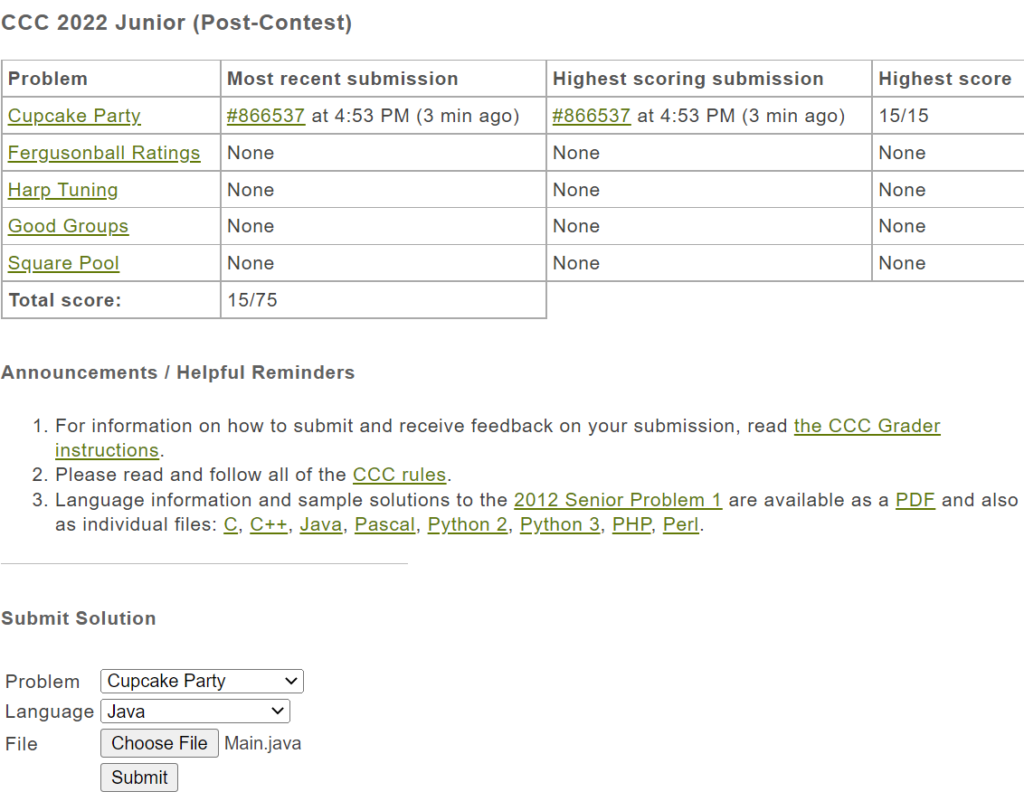
The problem list summarizes your solutions submitted for each problem, your score, and your total score for that competition round. Below it are rules and reminders – take some time to read through these, although some of that information is covered in our blog posts like this one. At the bottom, there is a form for submitting answers.
Problem Format
Clicking on a problem opens a description of it in pdf format. During work on a problem, you should probably keep the description open in one browser tab or window, and the submission page open in another. The description explains the scenario your solution program must solve, and gives exact details of how the data will be formatted for the program (input), and how the program should deliver the results (output).
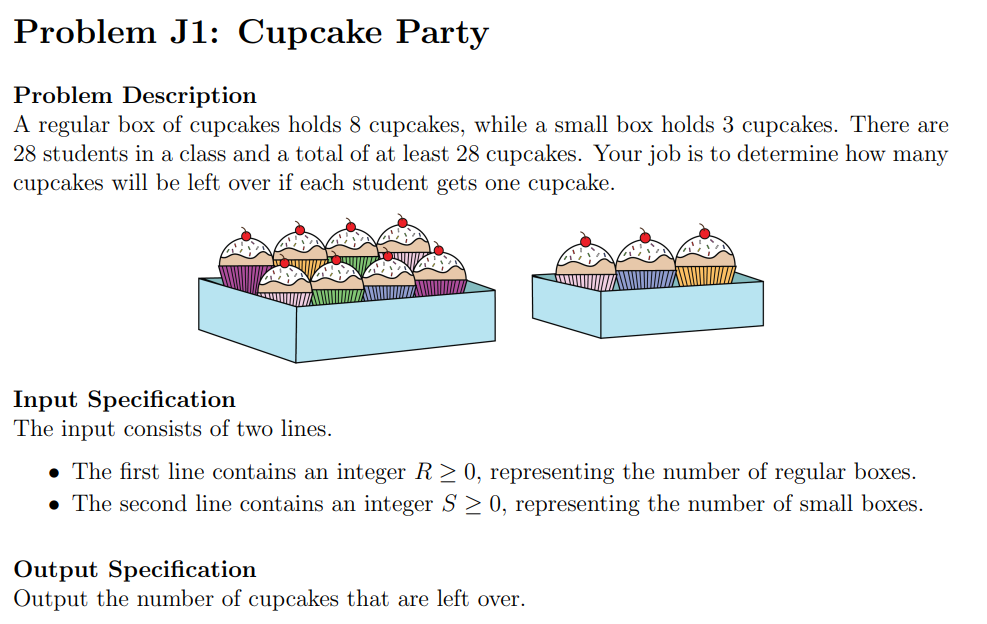
The goal for every problem is to write a program that analyzes data, sometimes in very clever ways, to find the answer to a question. These questions are based on real life scenarios, like how to distribute boxes of cupcakes or build the best swimming pool. The official event presents 5 problems, and competitors have 3 hours to try to write a different program for each one. Outside of the big event, you can work on any problem you like over any length of time as you improve your logic skills and coding speed through practice.
Here’s a complete Java program that solves the first problem from 2022’s Junior Division contest:
import java.util.*; import java.io.*; public class Main { public static void main(String[] args) throws Exception { Scanner in = new Scanner(System.in); int reg = in.nextInt(); int sm = in.nextInt(); in.close(); int ans = reg * 8 + sm * 3 - 28; System.out.println(ans); } }
You may notice, this code isn’t very long, and almost all of it is part of a “standard CCC framework”. Competitors only need to learn this “framework” once, since other solutions use the same format to handle input and output.
One thing to remember with the CCC is that each time you submit a Java solution, it must be in a class called Main, and a file named Main.java. Once you’re finished with a problem and ready to move on to another one, you can rename it to whatever you like for your own records. Of course, the next one will also need to be called Main.java while you work on it, so take your time and don’t get mixed up between your solutions.
Write any solution to get the data in the exact format specified for the problem. The Scanner
class in Java is a convenient tool to easily read data. It’s created with System.in
as an input to allow the program to read from “standard input”, which allows the website’s automated testing to provide the data to your program. Then, every call to in.nextInt()
reads another integer from the input, progressing through the data in exactly the order the problem specified. This works regardless of whether the integers are on one line or spread across many lines. In the above example, only two input numbers were needed, but this can be used as many times as necessary to get all the data.
For full credit, each submitted program must work for any data set it reads and draw a correct conclusion. Multiple data sets will be submitted to each program (by running your program multiple times), so this is not something where you can pre-calculate a numerical answer. The program itself must have the correct logic to compute the right answer for any data, within the limitations described in a problem. The logic needed varies from simple math calculations, to simulating a complex process the problem describes, to considering which combination of different possible actions in a hypothetical situation is best to achieve some goal.
Programs must also run within a time limit of 1-4 seconds. On easier problems, this isn’t a concern at all, but harder problems require efficient algorithms to receive full credit. This is one place where more advanced computer science skills covered in [CORE 6] (formerly CS02) and competition prep classes become very valuable! Luckily, you can still get a good score in Junior division without too much advanced training. You can use C++, Java, Python, and other languages, but C++ or Java are highly recommended. (They’re also very similar to each other!)
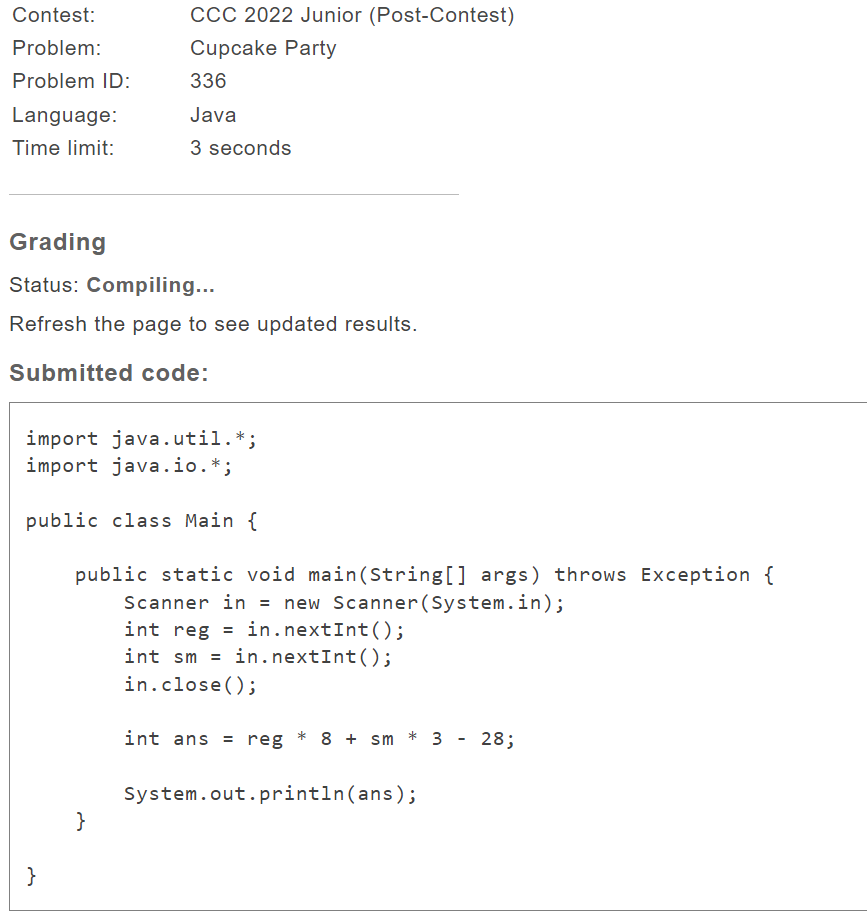
Once you’ve completed the program, you should test it by running the code and inputting sample data yourself. If it seems to be working, submit it through the grading site! One quirk of the CCC site is it requires refreshing the web page to update the results as the automatic grading system runs your submitted program multiple times with different data sets. After refreshing, color coded results will show for which data sets your program gave a correct answer (green), an incorrect answer (red), or hasn’t tested yet (yellow).
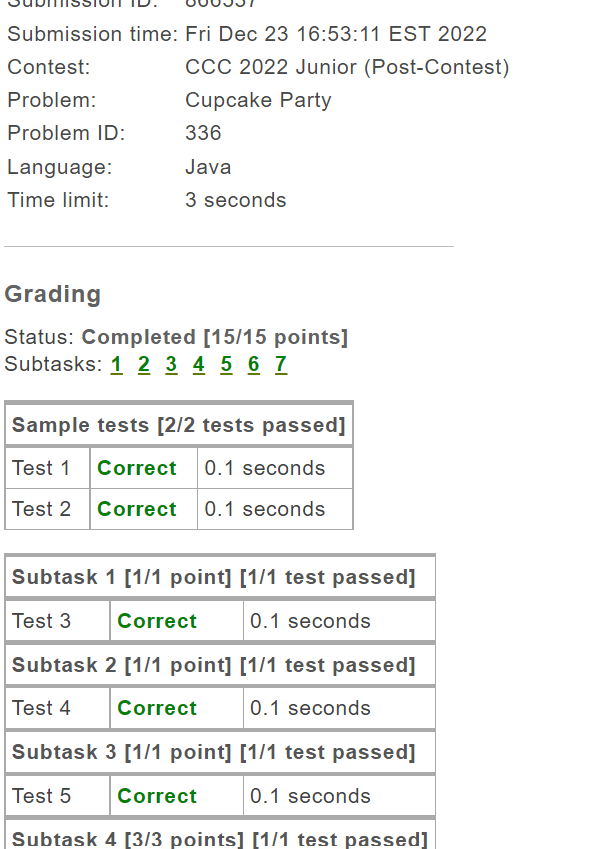
It’s important to remember you can try submitting a solution to a problem multiple times – the CCC allows up to 50 submissions for each, which will almost never be an issue during a contest event. You’re not expected to instantly know the answer and get it right on the first try! Instead, spend time developing, testing, and refining your program to solve as many test data sets as possible, even if you have to edit it quite a few times. Just like when you debug code for classwork or other projects, if your program isn’t working in all cases, you’ll have to think about what kinds of data you might not have accounted for, and trace through your code to see where it might not be taking the right steps for the data.
Scoring
Each problem is worth 15 points. Remember, your program is run multiple times with different test data each time. Points are divided up among different groups of test data called “subtasks”. For most problems, each subtask may have different restrictions on what the data can contain, which makes it easier for the program to analyze. This allows you to get partial credit even for solutions that may be inefficient or use logic that only works some of the time.
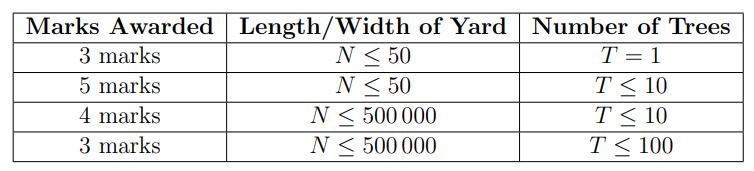
For instance, in the “Square Pool” problem whose subtasks are shown above, over half the credit is guaranteed to have data where a grid (representing someone’s back yard) is only 50×50 – a relatively small amount of spaces for a program to check directly. Nearly full credit can be obtained for a solution that relies on the number of trees in the yard being very small. Making the choice to write a program that only solves some subtasks at first can be a good contest strategy if you’re not sure how to solve them all. Remember, you can always modify the program to handle more subtasks later.
Note that grading is completely objective, based on whether your program produced exactly the correct answer for each test case, and whether it finished running in time. This means you don’t need to conform to any specific coding style or someone else’s judgement about the “best” solution, as long as it’s a correct solution. But it also means you need to be very careful setting up your program correctly – if a minor error in the technical details prevents your program from running correctly, you won’t get credit, even if the overall logic is very good. Luckily, it’s easy to avoid this by practicing setting up and submitting problems ahead of time.
The total score for the whole contest round is simply the total number of points across all 5 problems, with a maximum of 75. In the 2022 Junior contest, students with 67 points or more appeared on the Honor Roll, meaning you can solve the easiest 4 problems and only get half credit on the last, and still be publicly recognized for your achievement!
Your Coding Environment
To create a working Java program for each problem, you’ll need somewhere to write and test Java code. One easy place to do this is at https://replit.com/languages/java10. Replit is a handy website where small programs in many different languages can be run completely in your web browser. It doesn’t have as many features as a full scale IDE (Integrated Development Environment), but it does have a “console” where input and output can be handled, which is all you really need to create CCC solutions.
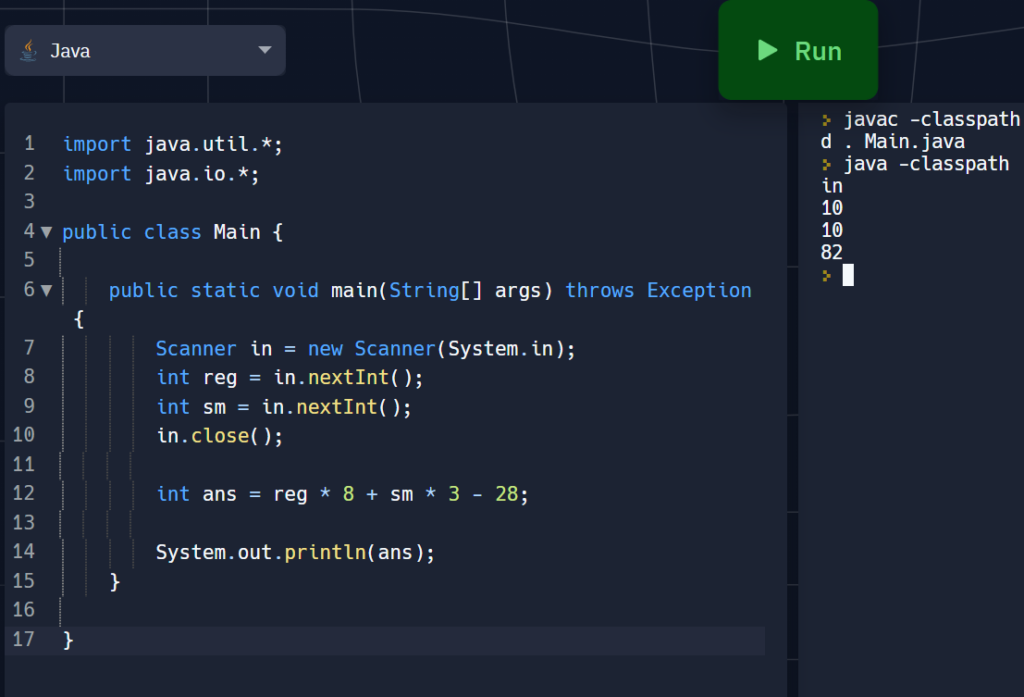
In the left panel, you can write your Java code, complete with syntax highlighting and other formatting tools you’re used to in other editors. Once it’s ready, click “Run”, and on the right you’ll see some startup commands. At that point the cursor will be sitting on a blank line, which can be confusing. Don’t worry, the program probably isn’t stuck, it’s just waiting for your input! You can type in the input numbers (10 and 10 in the example above) and press enter to generate the output (82). Here’s an extra tip: Instead of manually typing in a long set of sample data every time you test your program, just write it in a plain text program (like Notepad or TextEdit) once, copy it, and right click in the replit window to paste your data – this even works if the data is many lines long.
After you’ve tested in replit, you’ll still need to submit your program from a file saved on your computer. Just copy-paste your code into a text editor, save it as “Main.java”, and submit that file to the CCC grader! Saving it your computer’s desktop is often easiest.
You could also use a full scale IDE. KTBYTE [CORE 5b] students and above (formerly CS01b) are accustomed to Eclipse, a free professional grade Java editor which you can download at https://www.eclipse.org/downloads/ You will also need to install the Java JDK (Java Development Kit) from https://www.oracle.com/java/technologies/downloads/#java17 before installing Eclipse. We recommend setting this up on your computer ahead of time, although students without their own laptops can use KTBYTE’s virtual machines if they attend the contest event on February 15.
Contest Rules
The CCC is an individual contest. Each participant must do their own work during the actual contest event. You are allowed to look at online documentation for your programming language – for instance, names of methods for the Scanner class discussed above – but not tutorials, suggestions, or other discussion of problems or problem solving methods. Academic integrity is extremely important, and KTBYTE will not welcome back students who violate these rules!
The CEMC
Remember, KTBYTE doesn’t run this or other national programming contests ourselves, and we have no power over the problems or the scoring system. We are happy to provide access to the contest for our students and give advice, but the real credit for this contest goes to the Centre for Education in Mathematics and Computing (CEMC) at the University of Waterloo. The organization organizes, produces, and distributes learning and contest materials to thousands of students each year, including new CCC content annually. We’re very thankful for their work and the work of other IOI-style contest organizers to provide students with achievement opportunities in computer science!
Coming Soon
In our next few posts, we plan to go over:
- A line-by-line breakdown of the CCC “starter code” and a couple example solutions from recent contests.
- A look at installing the most recent versions of Java and Eclipse at home.
- A discussion of which classes help prepare for these kinds of contests.
Please leave a comment or get in touch with us via the contact info on our front page if you have questions about the CCC or would like to see posts about any other topics! And remember to stay tuned to our newsletter for additional announcements on the CCC, USACO, and other contests and activities.
Update: The 2023 Contest Is In-Person
In January, the CCC organizers clarified that this year’s contest will only be conducted in-person under the supervision of a registered school, unlike last year where students were able to participate online from home. We apologize for not clarifying the situation in the original version of this post.
KTBYTE will be hosting students in the Boston area as well as in Hong Kong for the event on February 15. This does require registering ahead of time, so please let us know if you would like to attend if you haven’t already!
Some students have also arranged to have their local schools proctor the contest for them. If you’re not close to our offices and weren’t able to arrange to take the contest this year at a local school, remember the contest is given annually, so stay in touch with your school’s math, computer science or guidance department to see if they might be able to host the contest next time!